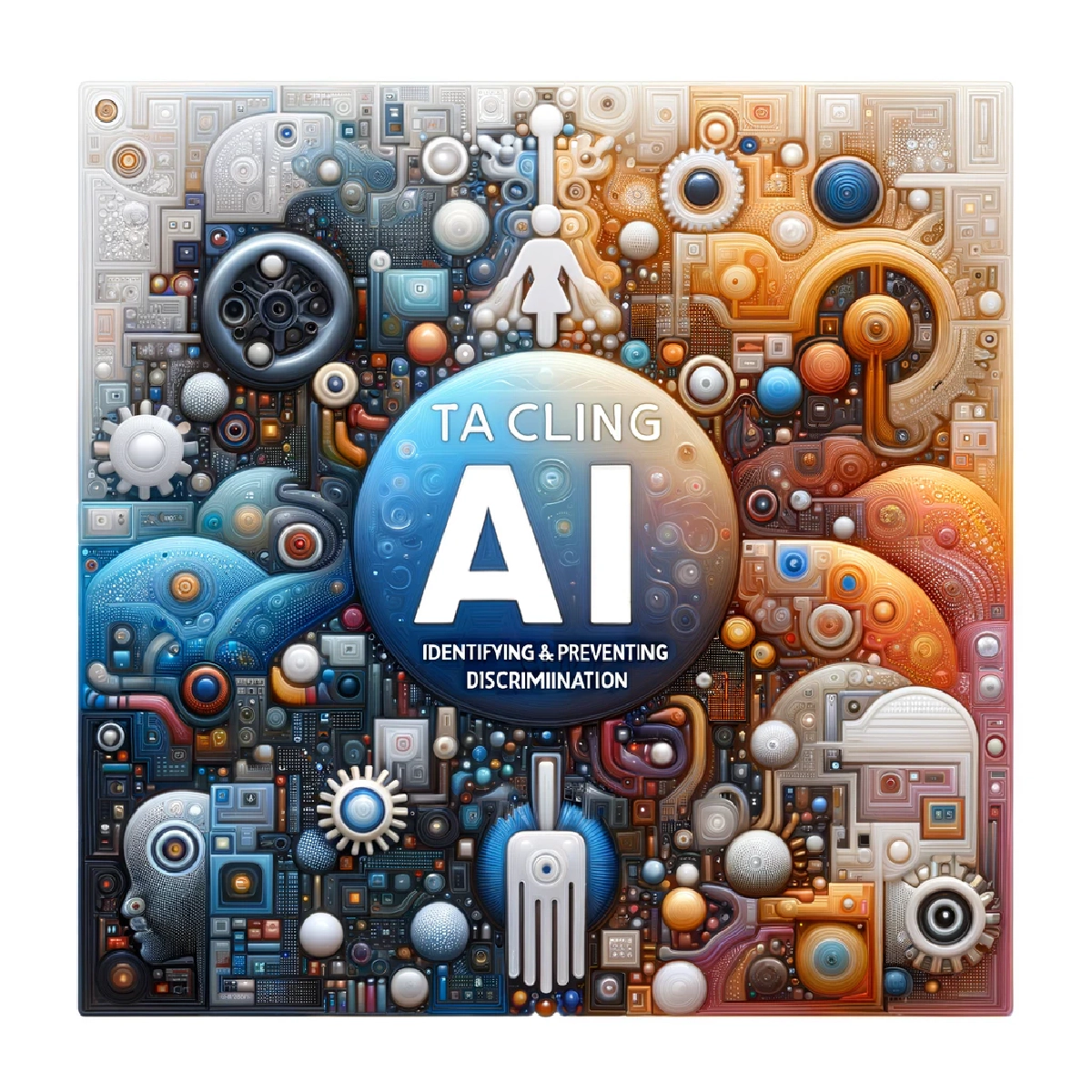
Tackling AI Bias: Identifying & Preventing Discrimination
13 Min read
Artificial intelligence (AI) has the potential to revolutionize numerous industries, but it is not without its pitfalls. …
React and Vue are two of the most popular JavaScript frameworks used in web development. Both frameworks are powerful and widely-used, but they have different use cases, areas of application, and philosophies. This article will compare React and Vue, covering their history, community, efficiency in updating UI, and other key features. Additionally, it will provide code snippets and diagrams to help developers understand the differences between the two frameworks. The article also provides factors to consider when choosing between the two frameworks, and their future in web development. This article will be a useful resource for developers and organizations looking to understand the pros and cons of React and Vue in web development.
Let's get started!
React and Vue are both popular JavaScript libraries for building user interfaces.
React, which was created and is maintained by Facebook, is a library for building reusable UI components. It uses a virtual DOM (Document Object Model) to efficiently update the UI in response to changes in data. React is often used for large and complex web applications, and it is also commonly used for building mobile apps with the help of React Native.
Vue, on the other hand, is a progressive JavaScript framework for building user interfaces. It was created by Evan You in 2014 and it’s maintained by an open-source community. Vue also focuses on building reusable UI components, but it has a simpler and more approachable syntax than React.
You can learn more about React on the official website and the React documentation
You can learn more about Vue on the official website and the Vue documentation
React was first released in 2013 by Facebook. It was created by Jordan Walke, a software engineer at Facebook. React was initially used for building the user interface of Facebook’s news feed, and it was later released as an open-source project. Since then, React has become one of the most popular JavaScript libraries for building user interfaces, and it’s widely used by companies and individual developers.
Vue, on the other hand, was created by Evan You in 2014. It was initially released as a small project, but it quickly gained popularity among developers. Vue was designed to be a lightweight alternative to other JavaScript frameworks and libraries, and it has a simpler and more approachable syntax than React. Vue has also gained popularity among developers, and it’s widely used in a variety of contexts, including web development and building user interfaces for existing projects.
React has undergone several major updates since its initial release in 2013. In 2015, React Native was released, which allowed developers to use React to build mobile apps for iOS and Android. In 2016, React Fiber was announced, which aimed to improve the performance of React by re-implementing the algorithm that schedules updates to the virtual DOM. React Fiber was eventually released in 2017 as React 16.
In the case of Vue, after its initial release in 2014, it has undergone several major updates, Vue.js 2.0 was released in 2016 which brought a lot of improvements and new features. With the release of Vue 3.0 in 2020, it has improved its performance and developer experience. Vue 3.0 also introduced a new composition API, which allows developers to write more flexible and expressive code.
React and Vue continue to evolve, with new features and improvements being added regularly. The communities behind each library are active and constantly working to improve the libraries and provide new resources for developers.
You can learn more about the history of React on the React Wikipedia page
You can learn more about the history of Vue on the Vue Wikipedia page
React is maintained by Facebook, and a community of individual developers and companies. Facebook uses React to build its own products, such as the Facebook website and Instagram. They also provide resources and support for developers who use React to build their own apps.
Vue is maintained by an open-source community. The core team of Vue is led by Evan You, the creator of Vue. The community is composed of developers from all around the world who contribute to the development of Vue. They also provide resources and support for developers who use Vue to build their own apps.
Both React and Vue are open-source projects, which means that anyone can contribute to their development. Developers can submit bug reports, feature requests, and code changes through the respective GitHub repositories.
You can learn more about the React team and community on the React team page and the React GitHub repository
You can learn more about the Vue team and community on the Vue team page and the Vue GitHub repository
Read more about how React can improve web development process
React uses a virtual DOM (Document Object Model) to efficiently update the UI in response to changes in data. The virtual DOM is a lightweight in-memory representation of the actual DOM. When the state of the application changes, React updates the virtual DOM instead of the actual DOM. Then, it calculates the difference between the virtual and actual DOMs, and applies only the necessary changes to the actual DOM.
This process is known as reconciliation, and it allows React to only update the parts of the UI that have changed, rather than re-rendering the entire interface. This results in a smoother and more efficient user experience.
graph TD A[Real DOM] --> B[Virtual DOM] B --> C[Diff] C --> D[Changes applied to Real DOM]
Keep in mind that Vue also uses a virtual DOM but it works a bit differently, Vue uses a template-based syntax and a reactivity system to manage the state of the application, which allows it to efficiently update the UI in response to changes in data.
The use of a virtual DOM in React allows for efficient updates to the user interface. When the state of the application changes, React updates the virtual DOM and then calculates the difference between the virtual and actual DOMs. It then applies only the necessary changes to the actual DOM, rather than re-rendering the entire interface. This results in a smoother and more efficient user experience.
Additionally, React also allows for the use of a component-based structure, which allows for the separation of concerns and ease of management of the state of the application. This helps in achieving efficient updates to the user interface, as the component that needs to be updated can be easily identified and updated.
In the case of Vue, the reactivity system and template-based syntax also allow for efficient updates to the user interface. Vue’s reactivity system allows it to track the changes in the state of the application, and it updates the necessary parts of the user interface accordingly.
graph LR A[State Changes] --> B(Virtual DOM updated) B --> C(Diff calculated) C --> D(Changes applied to Real DOM)
React has a large and active community of developers from all around the world. This community provides a wealth of resources and support for developers who use React to build their own apps. There are many tutorials, documentation, and online forums that provide help and guidance on using React. Additionally, there are many third-party libraries and tools that have been created by the community, which can be used to extend the functionality of React.
The large and active community also means that React is continuously evolving, new features and improvements are being added regularly. This helps ensure that React remains relevant and up-to-date with the latest developments in web development.
You can learn more about the React community on the React community page and join the React community on GitHub
Vue has a simpler and more approachable syntax than React. Vue’s template-based syntax allows developers to write templates using regular HTML, which makes it easier to learn for developers with experience in other frameworks or template languages. Additionally, Vue’s reactivity system allows developers to easily manage the state of the application and efficiently update the UI in response to changes in data.
The simplified syntax and reactivity system of Vue make it a good choice for building small to medium-sized web applications and building user interfaces for existing projects. It’s also suitable for developers who are new to front-end development or are looking for a simpler alternative to other JavaScript frameworks.
The following code snippet shows a simple example of a Vue component that displays a message when a button is clicked:
<template>
<div>
<button @click="showMessage = true">Show Message</button>
<p v-if="showMessage">Hello, Vue!</p>
</div>
</template>
<script>
export default {
data() {
return {
showMessage: false
}
}
}
</script>
This example uses Vue’s template-based syntax, which allows developers to write templates using regular HTML. The v-if
directive is used to conditionally render the message, and the @click
event listener is used to update the value of the
showMessage data property.
The following code snippet shows a simple example of a React component that displays a message when a button is clicked:
import React, { useState } from 'react';
function Example() {
const [showMessage, setShowMessage] = useState(false);
return (
<div>
<button onClick={() => setShowMessage(true)}>Show Message</button>
{showMessage && <p>Hello, React!</p>}
</div>
);
}
export default Example;
This example uses React’s component-based structure and JSX syntax. The useState hook is used to manage the component’s state, and the onClick event listener is used to update the value of the showMessage state variable.
As you can see, both examples achieve the same functionality, but the Vue
example is shorter, more readable and less
verbose than the React one. This illustrates Vue’s simpler and more approachable syntax and how it can be easier to
learn for developers with experience in other frameworks.
Vue is particularly well-suited for building small to medium-sized web applications. Its simpler and more approachable syntax, as well as its reactivity system, make it easy to learn and use for developers with experience in other frameworks or template languages. Additionally, Vue’s small size and minimal overhead make it well-suited for small to medium-sized web applications that don’t require the complexity and scalability of larger frameworks.
Vue also offers official libraries and tools that can easily be integrated into a project, such as Vue CLI, Vue Router and Vuex, which can help developers to scaffold and build their applications quickly and efficiently.
You can learn more about building small to medium-sized web applications with Vue on the Vue.js documentation
Vue is well-suited for a variety of use cases in web development, particularly for building user interfaces for existing projects. Its simplicity and ease of use make it a good choice for small to medium-sized web applications that don’t require the complexity and scalability of larger frameworks. Additionally, Vue’s reactivity system and template-based syntax make it easy to integrate with existing projects and to build user interfaces that are efficient and responsive to changes in data.
Vue is also suitable for building progressive web apps (PWA) and mobile apps using the Vue CLI and Vue Native.
Additionally, Vue’s small size and minimal overhead make it well-suited for projects with limited resources and for developers who are new to front-end development or are looking for a simpler alternative to other JavaScript frameworks.
React and Vue have slightly different design philosophies. React is primarily focused on building large-scale web applications, and it emphasizes a component-based structure and a unidirectional data flow. React also encourages the use of functional programming concepts and a minimalistic approach to the user interface.
On the other hand, Vue is focused on building user interfaces and is designed to be easy to learn and use for developers with experience in other frameworks or template languages. Vue’s template-based syntax and reactivity system allow for a more intuitive and expressive way of building user interfaces. Additionally, Vue’s design philosophy also emphasizes on flexibility and simplicity.
Both React and Vue are powerful and widely-used JavaScript frameworks, but they have different design philosophies and target use cases. React is more suitable for building large-scale web applications, while Vue is more suitable for building user interfaces for existing projects and small to medium-sized web applications.
One example of a difference in design philosophy between React and Vue is their approach to component architecture. React uses a component-based structure, where components are individual and self-contained units that manage their own state. This allows for a clear separation of concerns and makes it easier to manage the state of the application.
On the other hand, Vue uses a template-based syntax, where templates are written using regular HTML and directives are used to add interactivity and dynamic behavior to the user interface. This allows for a more intuitive and expressive way of building user interfaces and makes it easier to integrate with existing projects.
Another example is the way they handle the state management, React uses a unidirectional data flow, where the parent component passes down data to its child components through props and the child components can’t change the state of the parent component. Vue uses a reactivity system, where the state of the application is defined and tracked by Vue and it automatically updates the necessary parts of the user interface in response to changes in data.
To sum up, React’s design philosophy emphasizes on building large-scale web applications, with a component-based structure, unidirectional data flow, and functional programming concepts, while Vue’s design philosophy emphasizes on building user interfaces, with a template-based syntax, reactivity system, and simplicity.
Both React and Vue have large and active communities of developers from all around the world. These communities provide a wealth of resources and support for developers who use these frameworks to build their own apps.
React has a large number of tutorials, documentation, and online forums that provide help and guidance on using React. Additionally, there are many third-party libraries and tools that have been created by the community, which can be used to extend the functionality of React. The large and active community also means that React is continuously evolving, new features and improvements are being added regularly. You can learn more about the React community on the React community page and join the React community on GitHub
Vue also has a large and active community, which is relatively smaller than React’s. However, it is growing rapidly, and it provides a wealth of resources and support to developers. There are many tutorials, documentation, and online forums that provide help and guidance on using Vue. Additionally, there are many third-party libraries and tools that have been created by the community, which can be used to extend the functionality of Vue. The community is also continuously evolving, and new features and improvements are being added regularly. You can learn more about the Vue community on the Vue.js community page and join the Vue.js community on GitHub
If you would look to look fast through it, below is a table to compare key things
Feature | React | Vue |
---|---|---|
Scale and complexity | Suitable for building large-scale web applications with high degree of complexity | Suitable for building user interfaces for existing projects and small to medium-sized web applications |
Learning curve | Steep learning curve, may take longer for developers to become proficient in using it | Simpler and more approachable, easier to learn and use for developers with experience in other frameworks or template languages |
Syntax and structure | Component-based structure and JSX syntax | Template-based syntax and a reactivity system |
Community size and resources | Larger and more established community with more resources and support available | Rapidly growing community with a wealth of resources and support available |
Integration | More difficult to integrate into an existing project than Vue | Easier to integrate into an existing project, uses a template-based syntax and a reactivity system |
Flexibility | Less flexible, requires developers to structure their application using JSX | More flexible, allows developers to choose the way they want to structure their application, whether using a template-based syntax or JSX |
When choosing between React and Vue for your web development project, there are several factors to consider:
If you are building a large-scale web application that requires a high degree of complexity and scalability, React may be a better choice.
React has a steeper learning curve than Vue, and it may take longer for developers to become proficient in using it.
React uses a component-based structure and JSX syntax, while Vue uses a template-based syntax and a reactivity system. Developers who are more comfortable with HTML and templates may find Vue to be more approachable.
React has a larger and more established community than Vue, but Vue’s community is rapidly growing. This means that there are more resources and support available for React, but Vue is also well-supported.
Vue is easier to integrate into an existing project than React, as it uses a template-based syntax and a reactivity system that allows for a more intuitive and expressive way of building user interfaces.
Vue is more flexible than React, as it allows developers to choose the way they want to structure their application, whether using a template-based syntax or JSX.
Ultimately, the choice between React and Vue will depend on the specific requirements and goals of your project, as well as the preferences and skill level of your development team.
Read how we overcome challenges and build solid web dashboard for Cyanoguard - Cyanide Monitoring System
React is a powerful and widely-used JavaScript framework that is particularly well-suited for building large-scale web applications. Some of its key pros include:
Scalability and complexity: React is well-suited for building large-scale web applications that require a high degree of complexity and scalability. Its component-based structure and unidirectional data flow make it well-suited for applications that require a high degree of complexity and scalability.
Performance: React’s emphasis on functional programming concepts and a minimalistic approach to the user interface make it well-suited for building applications that require high performance and efficient updates to the user interface.
Large and active community: React has a large and active community of developers from all around the world. This means that there are many resources and support available for React.
However, React also has some cons, such as:
Steep learning curve: React has a steeper learning curve than other frameworks, which means that it may take longer for developers to become proficient in using it.
JSX syntax: React uses JSX syntax which is a combination of JavaScript and XML, it may be challenging for developers who are not familiar with it.
Vue is a versatile JavaScript framework that is well-suited for a variety of use cases in web development, particularly for building user interfaces for existing projects and small to medium-sized web applications. Some of its key pros include:
Easy to learn and use: Vue is designed to be easy to learn and use for developers with experience in other frameworks or template languages. Its simpler and more approachable syntax, as well as its reactivity system, make it easy to learn and use for developers.
Small size and minimal overhead: Vue’s small size and minimal overhead make it well-suited for projects with limited resources or MVPs and for developers who are new to front-end development or are looking for a simpler alternative to other JavaScript frameworks.
Flexibility: Vue is more flexible than other frameworks, as it allows developers to choose the way they want to structure their application, whether using a template-based syntax or JSX.
However, Vue also has some cons, such as:
React is a powerful and widely-used JavaScript framework that is particularly well-suited for building large-scale web applications. Its popularity and wide adoption in the industry have made it one of the most popular JavaScript frameworks for building web applications. React has a very active community and is continuously evolving, new features and improvements are being added regularly.
The future of React in web development looks bright, as it is expected to continue to be widely adopted by developers and organizations for building web applications. With the introduction of new features such as React Hooks and Suspense, React is becoming even more versatile and powerful, making it easier for developers to build complex and high-performance web applications.
Additionally, with the rise of technologies such as React Native and React VR, React is also being used to build cross-platform mobile and VR applications. This means that React developers will have more opportunities to work on a wide range of projects, including web, mobile, and VR applications.
Vue is a versatile JavaScript framework that is well-suited for a variety of use cases in web development, particularly for building user interfaces for existing projects and small to medium-sized web applications. Vue’s popularity and wide adoption in the industry have made it one of the most popular JavaScript frameworks for building web applications. Vue has a very active community and is continuously evolving, new features and improvements are being added regularly.
The future of Vue in web development looks bright, as it is expected to continue to be widely adopted by developers and organizations for building web applications. With the introduction of new features such as Vue 3, Vue is becoming even more versatile and powerful, making it easier for developers to build complex and high-performance web applications.
13 Min read
Artificial intelligence (AI) has the potential to revolutionize numerous industries, but it is not without its pitfalls. …
12 Min read
Quantum computing and artificial intelligence (AI) are two of the most revolutionary technological domains that are …
Looking for a solid engineering expertise who can make your product live? We are ready to help you!
Get in Touch