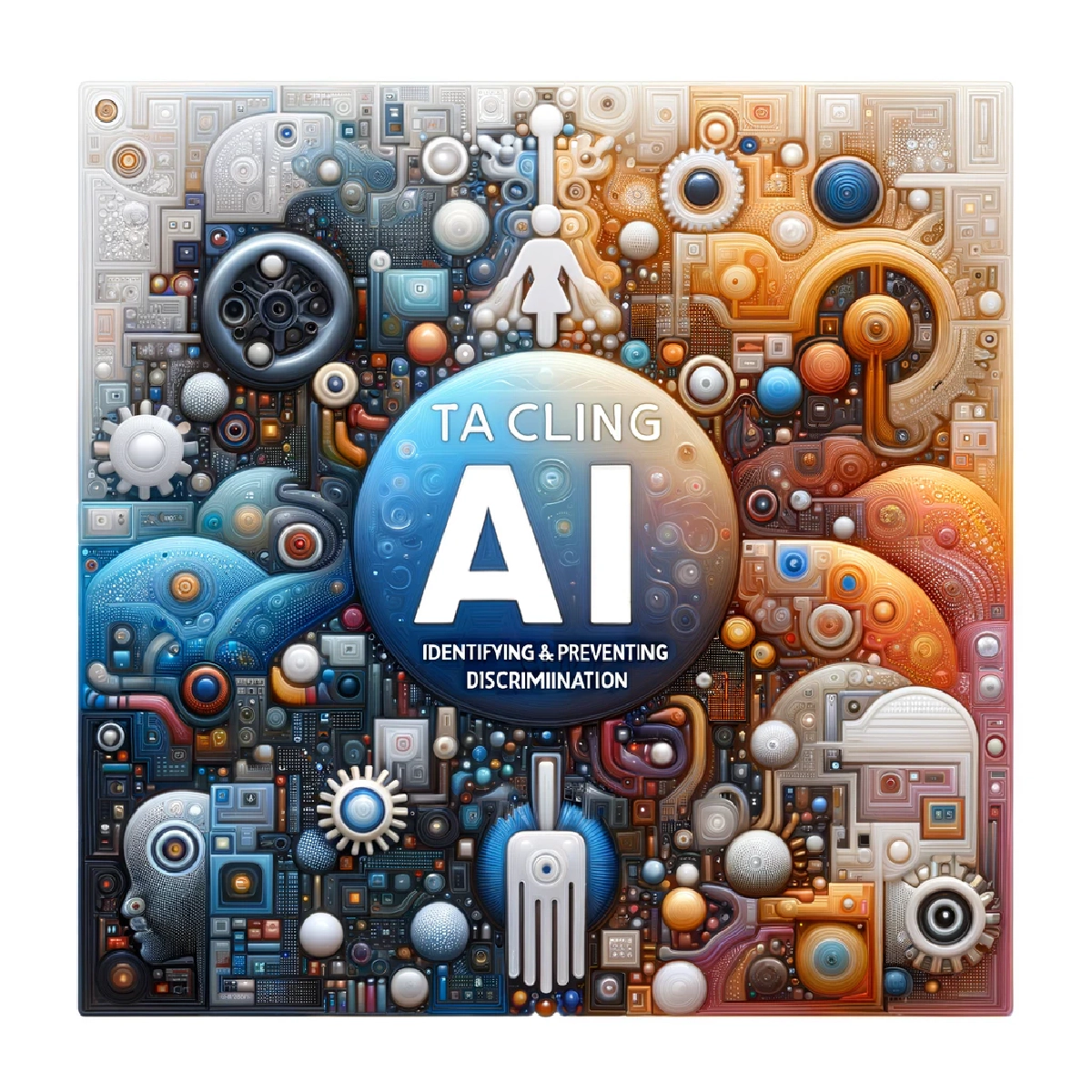
Tackling AI Bias: Identifying & Preventing Discrimination
13 Min read
Artificial intelligence (AI) has the potential to revolutionize numerous industries, but it is not without its pitfalls. …
Android application development can be a rewarding yet challenging process. To help you navigate these waters, here are the top 10 tips and best practices that will guide you towards creating a successful and efficient Android app.
Building an Android app can be a rewarding yet challenging process. To help you navigate these waters, here are the top 10 tips and best practices that will guide you towards creating a successful and efficient Android app.
How to develop an android app that would be attractive?? It pretty easy - create an engaging user interaction. The process begins with implementing responsive design elements. These elements are crucial for adapting the app’s layout and content to fit different device screens and orientations seamlessly. All visual elements should be aesthetically pleasing and functional, regardless of device specifications. Don’t forget to optimize touch feedback and gestures - this enhances the tactile experience for users. Effective touch feedback, such as subtle animations or haptic cues, provides immediate and intuitive responses to user inputs. This not only increases user satisfaction but also aids in reducing errors caused by unintended interactions.
Streamlining user journeys is key. This involves organizing content logically and avoiding overly complex menu hierarchies. Furthermore, reducing the necessity for user input can greatly enhance user satisfaction. Implement intelligent form technologies that can auto-fill information, thereby saving time and reducing user frustration. Simplifying user journeys not only typically leads to increase of the likelihood of user retention by minimizing friction and enhancing the overall user experience.
The visual appeal of an app is often the first thing a user notices. Modern design trends, such as flat design, minimalism, or material design, can capture users’ attention and make the interface enjoyable to navigate. Make sure color schemes and typography are consistent and create a harmonious visual flow throughout the app. This consistency helps in establishing brand identity and improves the intuitive use of the app, as users can easily predict the importance and function of different elements based on their visual treatment. Well-chosen colors and fonts not only make an app more attractive but also improve readability and user engagement.
What does it mean from the application development for android standpoint? User experience is first of all regular user testing and the integration of feedback into design updates. Conducting regular user testing sessions at different stages of the android mobile app development process allows for the identification and correction of usability issues before they affect a larger audience. These sessions can range from usability tests to beta testing with target users. Iterative design improvements are based on this strategy and feedback mechanisms can be integrated directly into the app. Ongoing loops of feedback and updates ensures that the app remains relevant, user-friendly, and above all, tailored to the needs and preferences of its user base.
The choice of android mobile app development framework greatly impacts the functionality and performance of an Android app. Developers must choose between native and cross-platform frameworks depending on their specific needs, such as performance requirements, target audience, and development timeline. Native frameworks provide optimized performance and better access to device-specific features, but at a higher cost and longer development time. Cross-platform frameworks offer faster deployment across multiple platforms but might come with limitations in performance and access to native APIs. Evaluating the performance of these frameworks and the support provided by their respective communities is essential for making an informed decision.
The most popular cross-platform options are Flutter and React Native nowadays. Each has pros and cons, for example Flutter works greatly when there designs looks similar between platforms. React Native, on the other hand - when there it should look natively to each platform. On the other hand - this leads to more bugs typically
The development environment can significantly affect the efficiency and quality of the app development process. Tools with strong debugging capabilities allow developers to quickly identify and fix errors, reducing downtime and improving the app’s reliability. Integrated design and development features can facilitate rapid prototyping and iterative testing, speeding up the development cycle and ensuring that the final product is well-polished. Developers should look for tools that support their specific workflow and enhance their productivity so that application development for android is interesting for both business and engineer.
How to develop android apps that provide a consistent user experience? Let’s talk about this using real examples.
Ensuring that an Android app provides a consistent user experience across a wide range of devices is a critical challenge due to the diversity in screen sizes, resolutions, orientations and manufacturers. Building adaptive layouts ensures that app’s interface adjusted to different screen dimensions, and the elements are presented optimally on each device. Testing on multiple screen sizes and resolutions is also crucial. Make sure that all users, regardless of their device type, experience the app as intended. User satisfaction and retention will drive a lot more revenue for you.
Android devices vary widely in terms of processing power, memory capacity, and available sensors. Optimizing an app to perform well across different hardware specifications can dramatically improve its accessibility and user experience. This involves coding the app to adapt its operation based on the device’s capabilities, such as simplifying graphics on lower-end devices or leveraging additional sensors on high-end devices for enhanced functionality. Handling diverse sensor inputs and ensuring the app utilizes these features effectively without draining the battery or overwhelming the processor is crucial for maintaining a smooth user experience.
Integrating quality assurance (QA) throughout the development process, starting from the initial design phases, is crucial for developing a high-quality Android app. Including QA early helps identify potential issues that could be costly to resolve later. This proactive approach to quality ensures that the app adheres to both functional and design standards from the start. Performing continuous integration and testing allows for the consistent monitoring and fixing of issues throughout the development cycle, which enhances the stability and reliability of the app.
Utilizing automated testing tools such as selenium or appium can significantly enhance the efficiency and coverage of testing. Unit testing frameworks help in validating each component or module of the application independently, ensuring that they function correctly before they are integrated into the larger system
Protecting user data should be a top priority in any Android application. Encrypting sensitive information is critical to safeguarding privacy and maintaining user trust. Implementing secure authentication mechanisms, such as OAuth or biometrics, adds an additional layer of security by ensuring that only authorized users can access their accounts.
graph LR A[Start] --> B{Encrypt Sensitive Data} B --> C[Implement Secure Authentication] C --> D[Regular Security Audits] D --> E[Apply Security Patches]
Security is not a one-time effort but an ongoing process. Regular security audits help identify and mitigate vulnerabilities before they can be exploited. Keeping the application and its libraries up-to-date with the latest security patches is crucial for defending against new threats.
Understanding and implementing Material Design principles is crucial for creating intuitive and delightful Android applications. Material components such as elevated surfaces, responsive animations, and transition patterns provide a coherent and immersive experience. Follow Material Design color guidelines and maintaining visual harmony as well as enhance the user interface readability.
Respecting Android’s interaction and interface standards ensures that the app feels familiar to users and integrates seamlessly with the device’s operating system. Utilizing platform-specific features wisely, such as notifications and widgets, can enhance functionality without compromising the app’s performance or user experience.
gantt title Example of debugging timeline dateFormat YYYY-MM-DD section Planning Phase Plan Testing :done, plan1, 2023-06-01,2023-06-03 Review Code :active, rev1, 2023-06-04, 3d section Execution Phase Execute Tests :exec1, after rev1, 5d Debugging Process :after exec1, 7d
Effective debugging is essential for a robust Android solution. Utilizing a variety of tools and simulators can help identify bugs across different devices and configurations. Integrate logging and diagnostic reporting within the app can provide valuable insights into its operation and help pinpoint areas for improvement.
Performance optimization should be a continuous focus throughout the development process. Identifying and resolving performance bottlenecks can lead to a smoother and more responsive app. Techniques such as profiling the app’s memory usage and optimizing database queries can significantly enhance its speed and efficiency.
Consistency in visual elements such as icons, typography, and UI components is key to a coherent user experience. Ensuring uniformity in these elements across the app helps in reducing cognitive load and making the interface more predictable and easier to navigate.
Creating a consistent navigational structure across the app helps users learn and remember pathways more effectively. Standardizing menu structures and ensuring predictable user paths can significantly enhance usability and user satisfaction.
Regular updates are essential to keeping an app relevant and functional over time. Scheduling regular evaluations and updates helps in addressing user needs, fixing bugs, and improving features. Preparing for platform updates and changes ensures that the app remains compatible with new OS versions and hardware.
Keeping the app content fresh and engaging is crucial for retaining users. Aligning updates with user feedback and analytics allows developers to understand user preferences and adapt the app accordingly. Introducing new features should be based on thorough testing and user demand to ensure they add value without complicating the user experience.
sequenceDiagram participant Dev as Developer participant App as Application Dev->>App: Evaluate Features App->>Dev: Gather User Feedback Dev->>App: Implement Updates App->>Dev: Deploy Changes
The purpose of a Minimum Viable Product (MVP) is to launch a product with enough features to attract early adopters and validate a product concept early in the development cycle. In short words - minimize the amount of up-front development effort and gather user feedback quickly to iterate and improve the product.
Main benefits are: high level of flexibility, high level of the engagement of the stakeholder, fast time to market. Additionally: easy management of changing priorities and a focus on user needs and value delivery.
Agile methodology is often considered better than Waterfall for projects where requirements are uncertain or likely to change. Agile practices allow iterative and incremental development. Teams can quickly adapt to changes and deliver working software more frequently. Waterfall, being a more linear and sequential approach, is less flexible. This typically makes it difficult to incorporate changes once the project has started.
The best use case - when the project involves complex, uncertain, or evolving requirements. It is suitable for projects where innovation, speed, and flexibility are important. Agile is also beneficial when the project stakeholders wish to be closely involved in the development process, providing frequent feedback and adjustments.
Sprint MVP refers to the most valuable and functional version of a product that can be delivered at the end of an Agile sprint. It focuses on delivering a specific set of functionalities that are enough to provide value to the user and meet the goals of the sprint, enabling quick testing and feedback. This concept aligns with Agile’s emphasis on rapid, incremental development and continuous improvement.
To make a good Android app, ensure a user-friendly design, maintain high performance and responsiveness, focus on solving a specific problem or need, and regularly update the app based on user feedback. Prioritize security and privacy to protect users.
Yes, it can be profitable to make Android apps, especially if they meet a unique need or serve a niche market. Profitability can be enhanced through in-app purchases, advertisements, subscriptions, and premium features.
The time to build an Android app can vary widely based on complexity, the features required, and team size. Simple apps might take a few months, while complex apps could require a year or more.
Kotlin is currently the best technology for android application development, recommended by Google. Java is also widely used. Technologies like Flutter and React Native are great for cross-platform app development.
Most Android apps are coded in Kotlin and Java. Kotlin is now the preferred language due to its conciseness, safety features, and alignment with modern programming standards.
Resources required include a skilled development team, Android Studio and SDK tools, a clear plan and design, APIs for third-party services, and testing devices or emulators. Access to user feedback and analytics tools is also important for ongoing improvement.
13 Min read
Artificial intelligence (AI) has the potential to revolutionize numerous industries, but it is not without its pitfalls. …
12 Min read
Quantum computing and artificial intelligence (AI) are two of the most revolutionary technological domains that are …
Looking for a solid engineering expertise who can make your product live? We are ready to help you!
Get in Touch