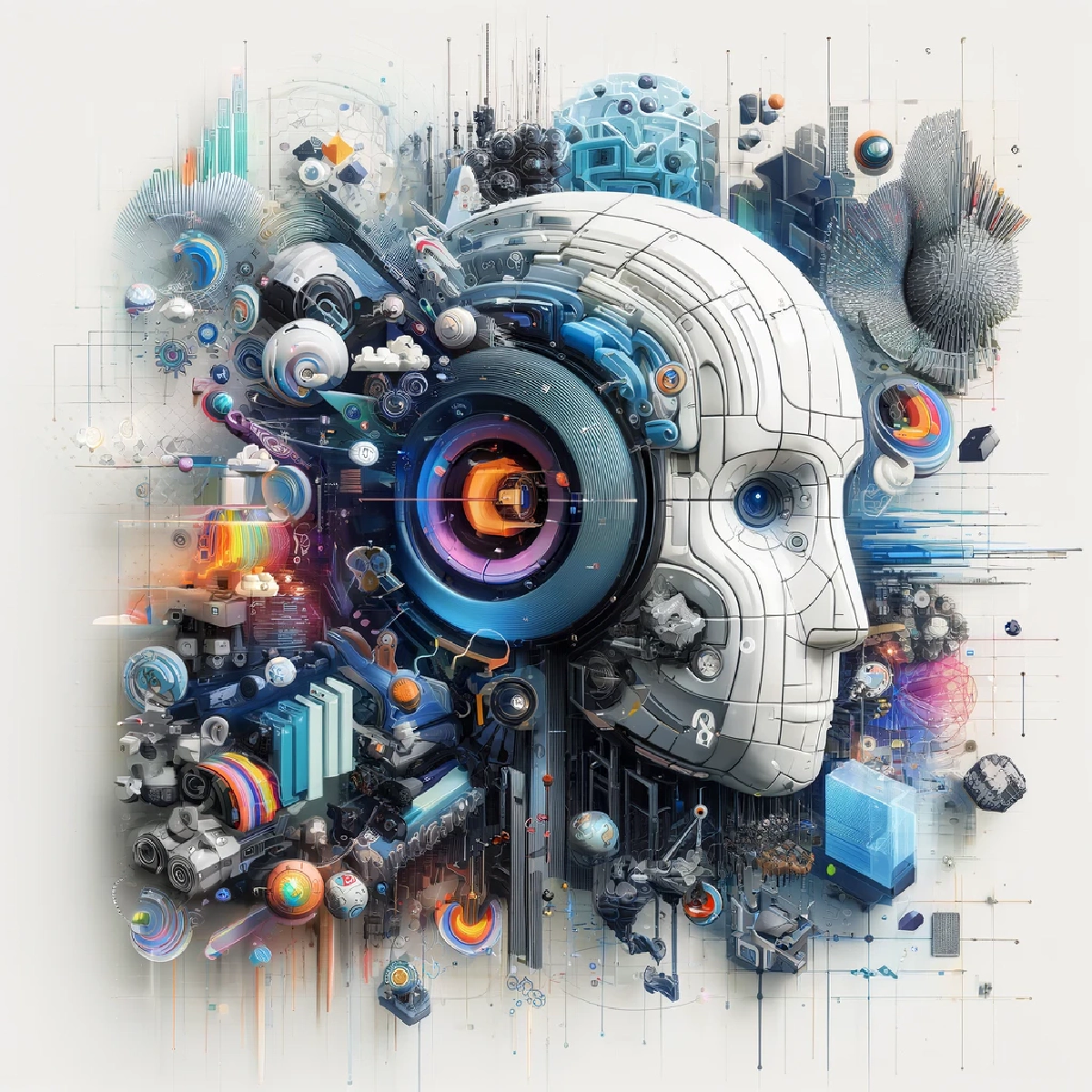
Deep Reinforcement Learning: Applications & Challenges
10 Min read
Deep Reinforcement Learning (DRL) stands at the forefront of AI, bridging the gap between the potential of artificial …
React is a powerful JavaScript library that has revolutionized the way developers build web applications. With its ability to create reusable UI components, improve performance with a virtual DOM, and ensure consistency with a one-way data flow, React has become a go-to choice for many developers. In this article, we will explore five ways in which React can improve your web development process, from saving time and effort to making your application easier to maintain and scale. Whether you are new to web development or an experienced developer, React has something to offer that can help you build better applications more efficiently.
Let's get started!
React is a JavaScript library for building user interfaces that was developed by Facebook in 2011. It has since become one of the most popular libraries for building web applications, and is used by companies such as Netflix, Airbnb, and Uber.
React has been used to develop many successful MVPs, including:
Airbnb: The popular vacation rental website was developed using React, and has since become one of the most successful startups in the world.
Dropbox: The cloud storage service used React to develop its MVP, which helped it to grow quickly and become a household name.
Asana: The project management tool used React to create its MVP, which has since become popular with teams and businesses around the world.
Uber: The ride-sharing service used React to develop its MVP, which has revolutionized the transportation industry and made it one of the most valuable companies in the world.
These MVPs demonstrate the power and versatility of React, and show how it can be used to create successful and scalable web applications.
Here are 5 ways in which React can improve your web development process:
One of the key features of React is its ability to create reusable components. These components can be used across your entire application, making it easier to maintain and scale.
Here is an example of a reusable button component in React:
import React from 'react';
const Button = (props) => {
return (
<button>{props.children}</button>
);
};
export default Button;
This button component can then be used in any other component in your application like this:
import Button from './Button';
const App = () => {
return (
<Button>Click me!</Button>
);
};
By using reusable components, you can save a lot of time and effort in the development process. It also helps to ensure that your application is consistent and easy to maintain.
Reusable components can also accept props, which are arguments that can be passed to the component. These props allow you to customize the component’s behavior and appearance. For example, you might have a button component that accepts a type
prop to determine whether it is a primary or secondary button:
import React from 'react';
const Button = (props) => {
return (
<button className={`btn btn-${props.type}`}>{props.children}</button>
);
};
export default Button;
This button component could then be used like this:
import Button from './Button';
const App = () => {
return (
<div>
<Button type="primary">Primary button</Button>
<Button type="secondary">Secondary button</Button>
</div>
);
};
Using reusable components can greatly improve your development process by saving time and effort, ensuring consistency, and making it easier to maintain and scale your application.
Our experience is illustrated at the chart below:
As you can see, it’s possible to have up to x2 speed of development, when reusing components
One of the key benefits of React is its virtual DOM, which is a lightweight in-memory representation of the actual DOM. When a developer makes changes to a React application, the virtual DOM is updated instead of the actual DOM. This can improve the performance of the application, as it reduces the number of DOM manipulations that need to be made.
The virtual DOM works by comparing the previous version of the virtual DOM with the new version after a change has been made. It then determines the minimum number of DOM manipulations required to update the actual DOM to reflect the changes, and performs those manipulations. This process is much faster than updating the DOM directly, as it reduces the number of costly DOM manipulations that need to be made.
In addition to improving performance, the virtual DOM also helps to make React applications more scalable. As the application grows in size and complexity, the virtual DOM can help to ensure that the performance of the application remains consistent.
Overall, the virtual DOM is a key feature of React that can help to improve the performance and scalability of web applications.
graph LR A[Actual DOM] -- Updates--> B[Virtual DOM] B -- Compares--> C[Updated Virtual DOM] C -- Updates--> A
More details described in the sequence diagram
sequenceDiagram Actual DOM ->> Virtual DOM: Updates Virtual DOM -->> Virtual DOM: Compares Virtual DOM -->> Virtual DOM: Determines minimum DOM manipulations required Virtual DOM -->> Updated Virtual DOM: Performs DOM manipulations Updated Virtual DOM ->> Actual DOM: Updates
In this sequence diagram, the Actual DOM sends an “Updates” message to the Virtual DOM, which in turn compares the previous version of the virtual DOM with the new version and determines the minimum number of DOM manipulations required to update the Actual DOM. The Virtual DOM then performs those DOM manipulations to create the updated Virtual DOM, which updates the Actual DOM.
Consistency is a critical aspect of web development, as it helps to ensure that the user interface (UI) behaves as expected and that data is handled correctly. Without consistency, it can be difficult to understand and predict the behavior of an application, leading to frustration and confusion for users.
React was designed with consistency in mind. It offers a number of features that help to improve consistency and make it easier to reason about the behavior of an application.
One of the key ways that React improves consistency is through the use of one-way data flow. In React, data flows in a single direction through the application, rather than being able to be modified by any component at any time. This helps to prevent inconsistencies by limiting the ways in which data can be modified, making it easier to understand and predict how the application will behave.
React also offers a number of developer tools, such as the React Developer Tools browser extension, that can help to improve consistency by making it easier to debug and understand the behavior of the application. These tools can help developers to identify and fix issues more quickly, leading to a more consistent and reliable experience for users.
gantt dateFormat YYYY-MM-DD axisFormat %m/%d title One-Way Data Flow in React section Parent Component Initialize props :done, des1, 2014-01-06,2014-01-08 Update props :active, des2, 2014-01-09, 3d Future task : des3, after des2, 5d section Child Component Receive props :crit, done, 2014-01-06,24h Update state :crit, done, after des1, 2d Pass props + state :crit, active, 3d Future task :crit, 5d Future task :2d Future task :1d
This Gantt chart diagram shows the one-way data flow in a React application. The “Initialize props” task represents the process of initializing the props in the Parent Component. The “Update props” task represents the process of updating the props in the Parent Component. The “Receive props” task represents the process of the Child Component receiving the props from the Parent Component, and the “Update state” task represents the process of the Child Component updating its own internal state. The “Pass props + state” task represents the process of the Child Component passing the props and state to a descendant component. This process illustrates the one-way flow of data in a React application, as the descendant component is not able to modify the props or state that it receives.
One of the key benefits of using react for web development is its intuitive API and developer tools. React’s API is designed to be easy to learn and use, making it accessible to developers of all skill levels. Its syntax is straightforward and familiar to developers who are already familiar with JavaScript, making it easy to get started with React.
React also provides a number of developer tools that can make it easier to develop, debug, and maintain applications. One of the most popular tools is the React Developer Tools browser extension, which allows developers to inspect and debug React components in their applications. This can be particularly useful for identifying and fixing issues, as well as for understanding the behavior of the application.
In addition to the React Developer Tools extension, React also provides a number of other tools and resources for developers. The React library includes a collection of reusable components that can save developers time and effort when building applications. The React Testing Library provides a set of tools for testing React components, making it easier to ensure that an application is working as intended. And the React CLI is a command-line interface tool that can be used to generate new React projects with a single command.
These tools can help to make the development process more efficient and streamlined, allowing developers to focus on building high-quality applications rather than on the details of the development process itself. Whether you are building a simple application or a complex web application, React’s API and developer tools can help you to build reliable and scalable applications with ease.
React is a popular JavaScript library for building user interfaces. It offers a number of benefits that can improve the web development process, including improved performance, reusable components, consistency with one-way data flow, an intuitive API, and developer tools.
One of the key ways that React improves performance is through the use of a virtual DOM, which reduces the number of DOM manipulations required to update the Actual DOM. This helps to ensure that the Actual DOM is always in sync with the virtual DOM, which in turn helps to improve the overall performance of the application.
React also makes it easier to build reusable components, which can save developers time and effort when building applications. These components can be easily shared and reused across different projects, making it easier to create consistent and scalable applications.
Consistency is an important aspect of web development, and React helps to improve consistency through the use of one-way data flow. This helps to prevent inconsistencies by limiting the ways in which data can be modified and making it easier to understand and predict how the application will behave.
React’s API is designed to be easy to learn and use, making it accessible to developers of all skill levels. It also provides a number of developer tools, such as the React Developer Tools browser extension, that can help to improve the development process and make it easier to debug and maintain applications.
Overall, React’s focus on performance, reusable components, consistency, and developer tools makes it a powerful and popular choice for web development. Whether you are building a simple application or a complex web application, React can help you to create reliable and scalable applications with ease.
CloudFlex is happy to support your development process!
10 Min read
Deep Reinforcement Learning (DRL) stands at the forefront of AI, bridging the gap between the potential of artificial …
10 Min read
How can artificial intelligence benefit healthcare? Artificial intelligence (AI) can significantly improve healthcare …
Looking for a solid engineering expertise who can make your product live? We are ready to help you!
Get in Touch